torchelie.recipes¶
Recipes are a way to provide off the shelf algorithms that ce be either used directly from the command line or easily imported in a python script if more flexibility is needed.
A recipe should, as much a possible, be agnostic of the data and the underlying model so that it can be used as a way to quickly try an algorithm on new data or be easily experimented on by changing the model
-
class
torchelie.recipes.recipebase.
Recipe
(call_fun, loader)¶ Basic recipe that iterates mutiple epochs over a dataset. That loop is instrumented through several configurable callbacks. Callbacks can handle events before and after each batch, before and after each epoch. Each batch is treated with a user supplied function that manipulates it and returns a dict that returns a state usable by the callbacks.
A recipe can be saved by calling its
state_dict()
member. All its hyper parameters and state will be saved so that it can restart, but requires the exact same setting of callbacks.You can register multiple objects to a Recipe with
register()
. If it has astate_dict()
member, its state will be saved into the recipe’s when calling the recipe’sstate_dict()
function. If it has a memberto()
, moving the recipe to another device will also move those objects.- Parameters
call_fun (Callable) – A function that takes a batch as an argument and returns a dict of value to feed the state
loader (Iterable) – any iterable (most likely a DataLoader)
-
cpu
()¶ Move a recipe and all its movable registered objects to cpu
-
cuda
()¶ Move a recipe and all its movable registered objects to cuda
-
load_state_dict
(state_dict)¶ Restore a recipe
-
modules
()¶ Iterate over all nn.Modules registered in the recipe
-
register
(name, value)¶ Register an object into the recipe as a member. Calling
recipe.register('foo', bar)
registers bar, and makes it usable throughrecipe.foo
.- Parameters
name (str) – member’s name
value – the object to register
-
run
(epochs)¶ Run the recipe for
epochs
epochs.- Parameters
epochs (int) – number of epochs
- Returns
The state
-
state_dict
()¶ - Returns
A state dict
-
to
(device)¶ Move a recipe and all its movable registered objects to a device
- Parameters
device – a torch device
-
torchelie.recipes.
TrainAndCall
(model, train_fun, test_fun, train_loader, test_every=100, visdom_env='main', checkpoint='model', log_every=10, key_best=None)¶ Train a model and evaluate it with a custom function. The model is automatically registered and checkpointed as
checkpoint['model']
, and put in eval mode when testing.Training callbacks:
Counter for counting iterations, connected to the testing loop as well
VisdomLogger
StdoutLogger
Testing:
Testing loop is in
.test_loop
.Testing callbacks:
VisdomLogger
StdoutLogger
Checkpoint
- Parameters
model (nn.Model) – a model
train_fun (Callabble) – a function that takes a batch as a single argument, performs a training step and return a dict of values to populate the recipe’s state.
test_fun (Callable) – a function taking no argument that performs something to evaluate your model and returns a dict to populate the state.
train_loader (DataLoader) – Training set dataloader
test_every (int) – testing frequency, in number of iterations (default: 100)
visdom_env (str) – name of the visdom environment to use, or None for not using Visdom (default: None)
checkpoint (str) – checkpointing path or None for no checkpointing
log_every (int) – logging frequency, in number of iterations (default: 10)
key_best (function or None) – a key function for comparing states. Checkpointing the greatest.
- Returns
a configured Recipe
-
torchelie.recipes.
TrainAndTest
(model, train_fun, test_fun, train_loader, test_loader, *, test_every=100, visdom_env='main', log_every=10, checkpoint='model', key_best=None)¶ Two nested loops, usually one for training and one for testing, but can serve other purposes. The model is automatically registered and checkpointed as
checkpoint['model']
, and put in eval mode when testing.Training callbacks:
Counter for counting iterations, connected to the testing loop as well
VisdomLogger
StdoutLogger
SeedDistributedSampler
Testing:
Testing loop is in
.test_loop
.Testing callbacks:
VisdomLogger
StdoutLogger
Checkpoint
- Parameters
model (nn.Model) – a model
train_fun (Callabble) – a function that takes a batch as a single argument, performs a training step and return a dict of values to populate the recipe’s state.
test_fun (Callable) – a function taking a batch as a single argument then performs something to evaluate your model and returns a dict to populate the state.
train_loader (DataLoader) – Training set dataloader
test_loader (DataLoader) – Testing set dataloader
test_every (int) – testing frequency, in number of iterations (default: 100)
visdom_env (str) – name of the visdom environment to use, or None for not using Visdom (default: None)
log_every (int) – logging frequency, in number of iterations (default: 100)
checkpoint (str) – checkpointing path or None for no checkpointing
key_best (function or None) – a key function for comparing states. Checkpointing the greatest.
-
torchelie.recipes.
Classification
(model, train_fun: Callable, test_fun: Callable, train_loader: Iterable[Any], test_loader: Iterable[Any], classes: List[str], *, visdom_env: Optional[str] = None, checkpoint: Optional[str] = None, test_every: int = 1000, log_every: int = 100)¶ Classification training and testing loop. Both forward functions must return a per-batch loss and logits predictions. It expands from
TrainAndTest
. Bothtrain_fun
andtest_fun
mustreturn {'loss': batch_loss, 'preds': logits_predictions}
. The model is automatically registered and checkpointed ascheckpoint['model']
, and put in eval mode when testing. The list of classes is checkpointed as well incheckpoint['classes']
.Training callbacks:
AccAvg for displaying accuracy
Log for displaying loss
ConfusionMatrix if len(classes) <= 25
ClassificationInspector
MetricsTable
Inherited training callbacks:
Counter for counting iterations, connected to the testing loop as well
VisdomLogger
StdoutLogger
Testing:
Testing loop is in
.test_loop
.Testing callbacks:
AccAvg
EpochMetricAvg
ConfusionMatrix if
len(classes) <= 25
ClassificationInspector
MetricsTable
Inherited testing callbacks:
VisdomLogger
StdoutLogger
Checkpoint saving the best testing loss
- Parameters
model (nn.Model) – a model
train_fun (Callabble) – a function that takes a batch as a single argument, performs a training forward pass and return a dict of values to populate the recipe’s state. It expects the logits predictions under key “preds”, and this batch’s loss under key “loss”.
test_fun (Callable) – a function taking a batch as a single argument then performs something to evaluate your model and returns a dict to populate the state. It expects the logits predictions under key “preds”, and this batch’s loss under key “loss”.
train_loader (DataLoader) – Training set dataloader
test_loader (DataLoader) – Testing set dataloader
classes (list of str) – classes name, in order
visdom_env (str) – name of the visdom environment to use, or None for not using Visdom (default: None)
test_every (int) – testing frequency, in number of iterations (default: 1000)
log_every (int) – logging frequency, in number of iterations (default: 100)
-
torchelie.recipes.
CrossEntropyClassification
(model, train_loader: Iterable[Any], test_loader: Iterable[Any], classes: List[str], *, lr: float = 0.001, beta1: float = 0.9, beta2: float = 0.999, wd: float = 0.01, visdom_env: Optional[str] = 'main', test_every: int = 1000, log_every: int = 100, checkpoint: Optional[str] = 'model', optimizer: typing_extensions.Literal[sgd, adamw] = 'adamw', n_iters: Optional[int] = None)¶ Extends Classification with default cross entropy forward passes. Also adds AdamW/SGD and CosineDecay schedule
Inherited training callbacks:
AccAvg for displaying accuracy
Log for displaying loss
ConfusionMatrix if len(classes) <= 25
ClassificationInspector
MetricsTable
Counter for counting iterations, connected to the testing loop as well
VisdomLogger
StdoutLogger
Training callbacks:
Optimizer with AdamW/SGD
LRSched with ReduceLROnPlateau
Testing:
Testing loop is in
.test_loop
.Inherited testing callbacks:
AccAvg
EpochMetricAvg
ConfusionMatrix if
len(classes) <= 25
ClassificationInspector
MetricsTable
VisdomLogger
StdoutLogger
Checkpoint saving the best testing loss
- Parameters
model (nn.Module) – a model learnable with cross entropy
train_loader (DataLoader) – Training set dataloader
test_loader (DataLoader) – Testing set dataloader
classes (list of str) – classes name, in order
lr (float) – the learning rate
beta1 (float) – AdamW’s beta1 / SGD’s momentum
beta2 (float) – AdamW’s beta2
wd (float) – weight decay
visdom_env (str) – name of the visdom environment to use, or None for not using Visdom (default: None)
test_every (int) – testing frequency, in number of iterations (default: 1000)
log_every (int) – logging frequency, in number of iterations (default: 1000)
n_iters (optional, int) – the number of iterations to train for. If provided, switch to a FlatAndCosineEnd scheduler
-
torchelie.recipes.gan.
GANRecipe
(G: torch.nn.modules.module.Module, D: torch.nn.modules.module.Module, G_fun, D_fun, test_fun, loader: Iterable[Any], *, test_loader: Optional[Iterable[Any]] = None, visdom_env: Optional[str] = 'main', checkpoint: Optional[str] = 'model', test_every: int = 1000, log_every: int = 10, g_every: int = 1) → torchelie.recipes.recipebase.Recipe¶ Experimental
Warning
GANRecipe() is experimental, and may change or be deleted soon if not already broken
.
Model Training¶
-
torchelie.recipes.trainandtest.
TrainAndTest
(model, train_fun, test_fun, train_loader, test_loader, *, test_every=100, visdom_env='main', log_every=10, checkpoint='model', key_best=None)¶ Two nested loops, usually one for training and one for testing, but can serve other purposes. The model is automatically registered and checkpointed as
checkpoint['model']
, and put in eval mode when testing.Training callbacks:
Counter for counting iterations, connected to the testing loop as well
VisdomLogger
StdoutLogger
SeedDistributedSampler
Testing:
Testing loop is in
.test_loop
.Testing callbacks:
VisdomLogger
StdoutLogger
Checkpoint
- Parameters
model (nn.Model) – a model
train_fun (Callabble) – a function that takes a batch as a single argument, performs a training step and return a dict of values to populate the recipe’s state.
test_fun (Callable) – a function taking a batch as a single argument then performs something to evaluate your model and returns a dict to populate the state.
train_loader (DataLoader) – Training set dataloader
test_loader (DataLoader) – Testing set dataloader
test_every (int) – testing frequency, in number of iterations (default: 100)
visdom_env (str) – name of the visdom environment to use, or None for not using Visdom (default: None)
log_every (int) – logging frequency, in number of iterations (default: 100)
checkpoint (str) – checkpointing path or None for no checkpointing
key_best (function or None) – a key function for comparing states. Checkpointing the greatest.
-
torchelie.recipes.classification.
Classification
(model, train_fun: Callable, test_fun: Callable, train_loader: Iterable[Any], test_loader: Iterable[Any], classes: List[str], *, visdom_env: Optional[str] = None, checkpoint: Optional[str] = None, test_every: int = 1000, log_every: int = 100)¶ Classification training and testing loop. Both forward functions must return a per-batch loss and logits predictions. It expands from
TrainAndTest
. Bothtrain_fun
andtest_fun
mustreturn {'loss': batch_loss, 'preds': logits_predictions}
. The model is automatically registered and checkpointed ascheckpoint['model']
, and put in eval mode when testing. The list of classes is checkpointed as well incheckpoint['classes']
.Training callbacks:
AccAvg for displaying accuracy
Log for displaying loss
ConfusionMatrix if len(classes) <= 25
ClassificationInspector
MetricsTable
Inherited training callbacks:
Counter for counting iterations, connected to the testing loop as well
VisdomLogger
StdoutLogger
Testing:
Testing loop is in
.test_loop
.Testing callbacks:
AccAvg
EpochMetricAvg
ConfusionMatrix if
len(classes) <= 25
ClassificationInspector
MetricsTable
Inherited testing callbacks:
VisdomLogger
StdoutLogger
Checkpoint saving the best testing loss
- Parameters
model (nn.Model) – a model
train_fun (Callabble) – a function that takes a batch as a single argument, performs a training forward pass and return a dict of values to populate the recipe’s state. It expects the logits predictions under key “preds”, and this batch’s loss under key “loss”.
test_fun (Callable) – a function taking a batch as a single argument then performs something to evaluate your model and returns a dict to populate the state. It expects the logits predictions under key “preds”, and this batch’s loss under key “loss”.
train_loader (DataLoader) – Training set dataloader
test_loader (DataLoader) – Testing set dataloader
classes (list of str) – classes name, in order
visdom_env (str) – name of the visdom environment to use, or None for not using Visdom (default: None)
test_every (int) – testing frequency, in number of iterations (default: 1000)
log_every (int) – logging frequency, in number of iterations (default: 100)
-
torchelie.recipes.classification.
CrossEntropyClassification
(model, train_loader: Iterable[Any], test_loader: Iterable[Any], classes: List[str], *, lr: float = 0.001, beta1: float = 0.9, beta2: float = 0.999, wd: float = 0.01, visdom_env: Optional[str] = 'main', test_every: int = 1000, log_every: int = 100, checkpoint: Optional[str] = 'model', optimizer: typing_extensions.Literal[sgd, adamw] = 'adamw', n_iters: Optional[int] = None)¶ Extends Classification with default cross entropy forward passes. Also adds AdamW/SGD and CosineDecay schedule
Inherited training callbacks:
AccAvg for displaying accuracy
Log for displaying loss
ConfusionMatrix if len(classes) <= 25
ClassificationInspector
MetricsTable
Counter for counting iterations, connected to the testing loop as well
VisdomLogger
StdoutLogger
Training callbacks:
Optimizer with AdamW/SGD
LRSched with ReduceLROnPlateau
Testing:
Testing loop is in
.test_loop
.Inherited testing callbacks:
AccAvg
EpochMetricAvg
ConfusionMatrix if
len(classes) <= 25
ClassificationInspector
MetricsTable
VisdomLogger
StdoutLogger
Checkpoint saving the best testing loss
- Parameters
model (nn.Module) – a model learnable with cross entropy
train_loader (DataLoader) – Training set dataloader
test_loader (DataLoader) – Testing set dataloader
classes (list of str) – classes name, in order
lr (float) – the learning rate
beta1 (float) – AdamW’s beta1 / SGD’s momentum
beta2 (float) – AdamW’s beta2
wd (float) – weight decay
visdom_env (str) – name of the visdom environment to use, or None for not using Visdom (default: None)
test_every (int) – testing frequency, in number of iterations (default: 1000)
log_every (int) – logging frequency, in number of iterations (default: 1000)
n_iters (optional, int) – the number of iterations to train for. If provided, switch to a FlatAndCosineEnd scheduler
Deep Dream¶
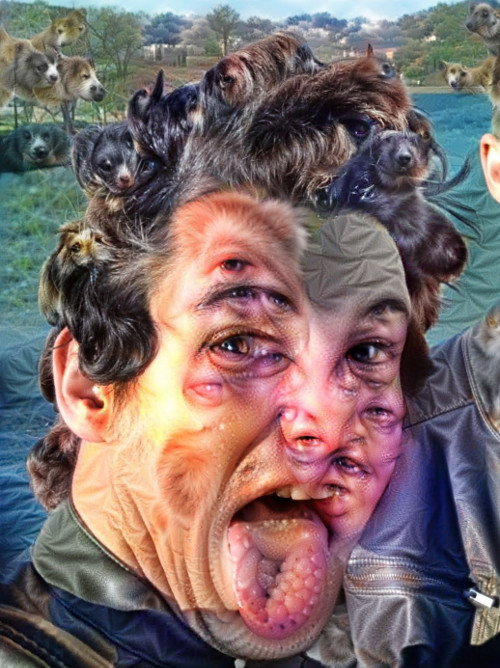
Deep Dream recipe.
Performs the algorithm described in https://ai.googleblog.com/2015/06/inceptionism-going-deeper-into-neural.html
This implementation differs from the original one: the image is optimized in Fourier space, for greater details and colors, the model and layers are customizable.
A commandline interface is provided through python3 -m torchelie.recipes.deepdream, and a DeepDreamRecipe is provided.
-
class
torchelie.recipes.deepdream.
DeepDream
(model, dream_layer)¶ Deep Dream recipe
First instantiate the recipe then call recipe(n_iter, img)
- Parameters
model (nn.Module) – the trained model to use
dream_layer (str) – the layer to use on which activations will be maximized
-
fit
(ref, iters, lr=0.0003, device='cpu', visdom_env='deepdream')¶ - Parameters
lr (float, optional) – the learning rate
visdom_env (str or None) – the name of the visdom env to use, or None to disable Visdom
Feature visualization¶
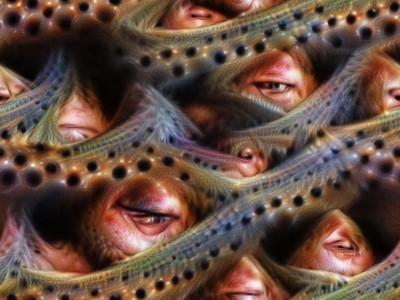
Feature visualization
This optimizes an image to maximize some neuron in order to visualize the features it captures
A commandline is provided with python3 -m torchelie.recipes.feature_vis
-
class
torchelie.recipes.feature_vis.
FeatureVis
(model, layer, input_size, *, num_feature=3, lr=0.001, device='cpu', visdom_env='feature_vis')¶ Feature viz
First instantiate the recipe then call recipe(n_iter, img)
- Parameters
model (nn.Module) – the trained model to use
layer (str) – the layer to use on which activations will be maximized
input_size (int, or (int, int)) – the size of the image the model accepts as input
num_feature (int) – the number of channels of the input image (e.g 1 for grey, 3 for RGB)
lr (float, optional) – the learning rate
device (device) – where to run the computation
visdom_env (str or None) – the name of the visdom env to use, or None to disable Visdom
-
fit
(n_iters, neuron)¶ Run the recipe
- Parameters
n_iters (int) – number of iterations to run
neuron (int) – the feature map to maximize
- Returns
the optimized image
Neural Style¶
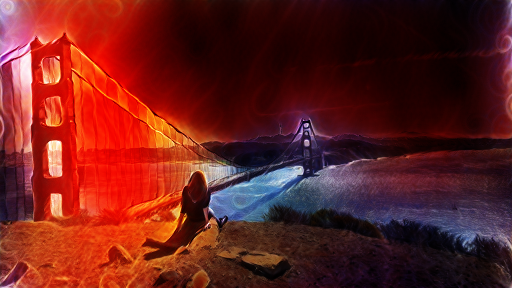
Neural Style from Leon Gatys
A commandline interface is provided through python3 -m torchelie.recipes.neural_style
-
class
torchelie.recipes.neural_style.
NeuralStyle
(device='cpu', visdom_env='style')¶ Neural Style Recipe
First instantiate the recipe then call recipe(n_iter, img)
- Parameters
device (device) – where to run the computation
visdom_env (str or None) – the name of the visdom env to use, or None to disable Visdom
-
fit
(iters, content_img, style_img, style_ratio, *, second_scale_ratio=1, content_layers=None, init_with_content=False)¶ Run the recipe
- Parameters
n_iters (int) – number of iterations to run
content (PIL.Image) – content image
style (PIL.Image) – style image
ratio (float) – weight of style loss
content_layers (list of str) – layers on which to reconstruct content
Deep Image Prior¶
Deep Image Prior as a command line tool is in this recipe, it provides super-resolution and denoising.
python3 -m torchelie.recipes.image_prior
for the usage message